Colour by code in Excel with VBA - Part 1
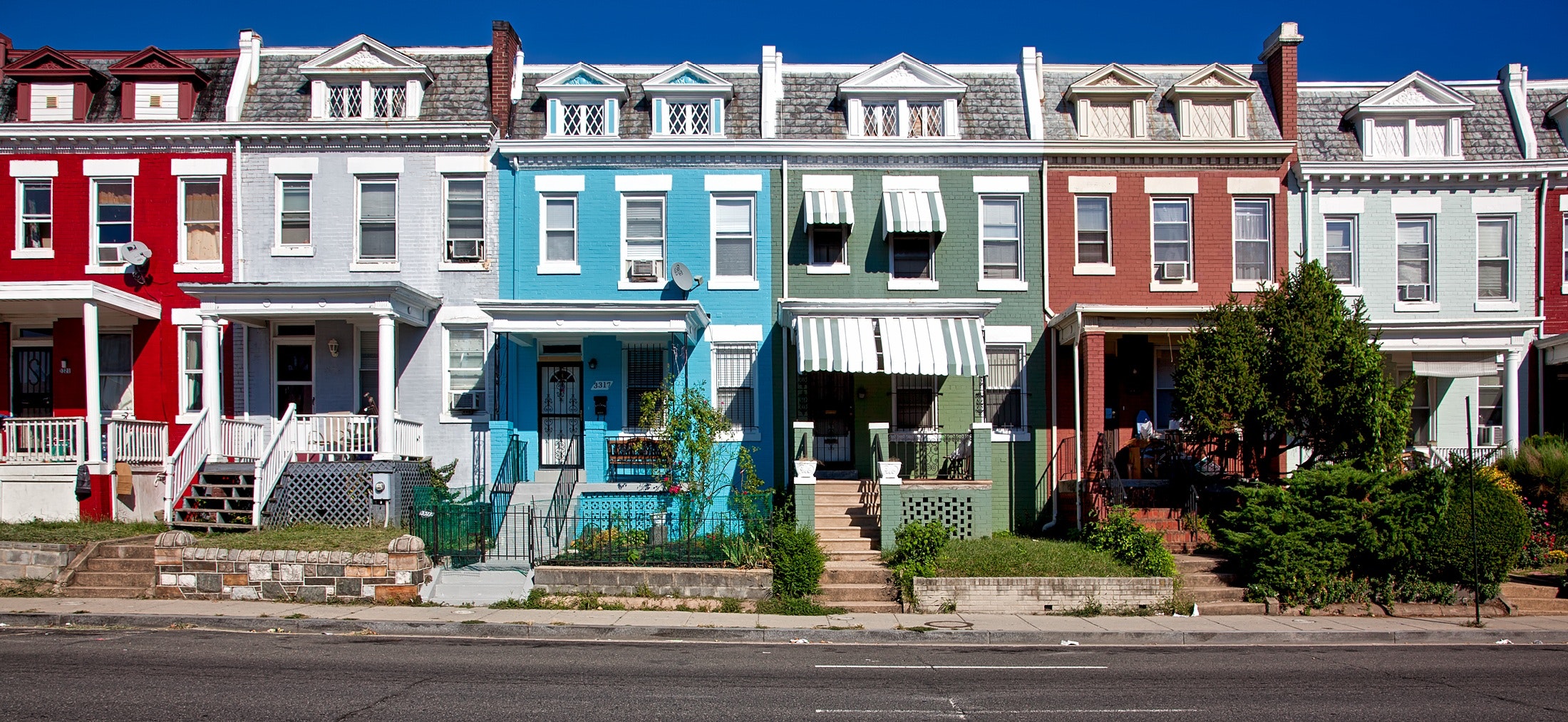
- 0 0
-
shares
Want a quick way to highlight cells by their content ? Read on!
Before:
After:
As of the writing of this article, Excel doesn't have much by way of separating out text fields by colour, despite having had a variety of numeric gradient formatting for a number of years.
RGB
All colours on screens are made of RGB pixels , from this you can mix any colour you like:
For example - Cyan is a mixture of Green and Blue, Purple a mixture of Red and blue, Grey is an even mix of all colours.
You can read up on it here : https://en.wikipedia.org/wiki/RGB_color_model
We can use these components and conditionally mix them to create our background colours.
ASCII
The next thing we need to know, is that every letter in the alphabet has a corresponding number - this is called an ASCII code. In the standard ASCII set there are 255 characters, which includes, numbers, letters, punctuation and special non-printing characters.
RGB colour in Excel is stored as a range of 0-255 for each 'pot' - if we just take the first, middle and last character from a string, and turn it into a colour, we get the below :
It works, but the colours are very dull. Clearly converting straight from ASCII to RGB isn't going to work.
So what now?
We just need to be smarter about how we're applying the numbers.
In the standard ASCII set , the 26 Uppercase, 26 Lowercase and 10 numbers only a quarter of the possible ASCII codes, and represent a limited range, therefore we're not getting sufficient variance from simply applying them directly to a colour mix.
Instead what we need to do, is sort them into sets ( Upper, Lower and Numeric ) , from there, we can determine set upper and lower boundaries for that set, and with that fixed, we can calculate a generic position within that ranger, and return a value between 0 and 1.
First, what are the bounds?
So we have uppercase, running from 65 - 90 , lower from 97 -122 and numeric from 48-57 .
Our first function - lets take the character as an input, then return a float of range of 0-1 depending on which category it falls in ( Upper, Lower or Numeric ) .
Here's the output - side by side with the original- already the colours are looking more defined.
Here's the code to return the factor float based on the cell text :
In the below chart - just looking at the first half of the ASCII range, you can see how it works :
Where it fits within a bracket ( upper / lower / numeric ) it returns 0-1 relative to that bracket, else it'll return 0-1 based on it's overall position in the range of 0-255.
Putting it all together
Now we have our conditional range - its' now time to put it into a procedure to apply to ranges.
In the above, it'll analyse the first, middle and last letter of cell to return the factor 0-1 , with a slight bias towards green.
There's also a conditional statement at the end, which, when specified, will assign a specific colour to a specific word, this is useful for generic columns with Y/N Yes/No True/False etc. ( Or names ) .
As an example of usage - here a quick currency char is spiced up by the automatic colours. Where it's based on content, and therefore deterministic - users will quickly be able to spot lines / items by colour ( for example, USD will always be Salmon coloured, GBP be Turquoise etc. )